A simple example demonstrating how we can add custom contact form in WordPress with validation and submitting the form using ajax.
Now a days any one can find number of WordPress plugins in the market which are simple and easy to use as a contact form, but still you need different ways to interact with your readers with customised forms and validations. Then there comes a need of customised forms to capture visitors informations or leads.
So we will be creating a simple contact form with fields like Name, Email, Phone, Location. You can add more fields according to your requirement. We will validate the form with javascript as well as php before inserting into database.
Also demonstrate how we can submit the form with a success page show after submission and how we can submit the contact from with out reloading the page using ajax.
Steps to create custom contact us form in WordPress without plugins
- Create HTML form with input fields
- Validate the form using JavaScript
- Create PHP function to submit form fields into Database Table.
Create HTML form with input fields
We will be adding a Contact Form in Side Bar as well as we will create page for Contact us form. So that in the custom contact us page we can have more content described and other information. You can also add the same form in a Plugin like Popup Maker and show when a user/visitor comes first time to your blog/Website.
Adding custom contact us page in WordPress
- In Dashboard, Go to Pages -> Add New
- Give a title for the contact form.
- Now navigate to the Text tab and add the HTML for input fields
- Preview and Publish your page.
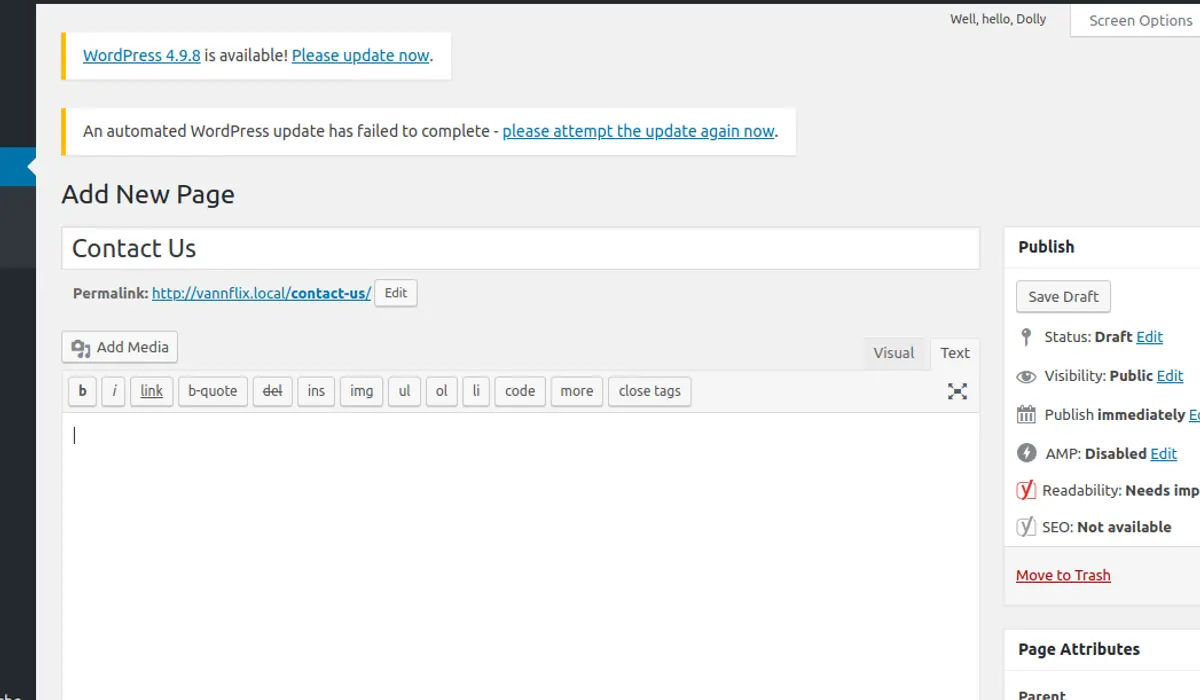
Adding custom contact us form in WordPress Website Sidebar
- In Dashboard, Go to Appearance -> Widgets
- Drag and Drop Custom HTML from the available widgets to your sidebar.
- Add a Title and Paste the HTML for contact form in the content and click save.
Adding custom contact us form in Popup Maker
- Install and Activate Popup Maker
- In Popup Maker Dashboard, click on add pop up
- Add Title for the Popup Maker.
- Click on Text tab and paste the HTML for contact form and save.
HTML Code for input fields in contact us page or PopupMaker
<form id="cstpop-jsform" class="form-control" action="../customer-information.php" method="POST" name="visitor_information">
<span>Fields marked with an * are required</span>
<span class="form-group col-md-12"><label for="name">Name *</label>
<input id="pop-name" class="form-control" name="name" type="text" placeholder="Full Name" /></span>
<span class="form-group"><label for="email">Email address *</label>
<input id="pop-email" class="form-control" name="email" type="email" placeholder="Enter email" aria-describedby="emailHelp" />
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small></span>
<span class="form-group"><label for="phone">Phone *</label>
<input id="pop-phone" class="form-control" type="text" name="phone" placeholder="Phone Number" /></span>
<span class="form-group"><label for="state">State *</label>
<select id="pop-state" class="form-control" name="state">
<option selected="selected" value="0">Select State</option>
<option value="andhra-pradesh">Andhra Pradesh</option>
<option value="delhi">Delhi</option>
<option value="goa">Goa</option>
<option value="jammu-and-kashmir">Jammu and Kashmir</option>
<option value="karnataka">Karnataka</option>
<option value="kerala">Kerala</option>
</select>
</span>
<span>
<button id="cstpop-jsbtn" class="btn btn-default" type="button" value="Submit">Submit</button>
</span>
</form>
<span id="pop-success"></span>
<!--HTML Code for Sidebar-->
<form class="form-control" action="../customer-information.php" method="POST" id="cst-jsform"name="customer_information">
<span>Fields marked with an * are required</span>
<span class="form-group"><label for="name">Name *</label><br>
<input id="name" class="form-control" name="name" type="text" placeholder="Full Name" /></span><br>
<span class="form-group"><label for="email">Email address *</label><br>
<input id="email" class="form-control" name="email" type="email" placeholder="Enter email" aria-describedby="emailHelp" /><br>
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small></span><br>
<span class="form-group"><label for="phone">Phone *</label><br>
<input id="phone" class="form-control" type="text" name="phone" placeholder="Phone Number" /></span><br>
<span class="form-group"><label for="state">State *</label><br>
<select id="state" class="form-control" name="state">
<option selected="selected" value="0">Select State</option>
<option selected="selected" value="0">Select State</option>
<option value="andhra-pradesh">Andhra Pradesh</option>
<option value="delhi">Delhi</option>
<option value="goa">Goa</option>
<option value="jammu-and-kashmir">Jammu and Kashmir</option>
<option value="karnataka">Karnataka</option>
<option value="kerala">Kerala</option>
</select></span><br>
<span>
<button class="btn btn-default" type="button" value="Submit" id="cst-jsbtn">Submit</button>
</span>
</form>
<span id="jsform-success" hidden="hidden"></span>
Validate the form using JavaScript
For this step we will add the script in our theme js file, or else you can add in any js file which your WordPress application is using.
Different methods to Submit custom contact us form in WordPress without plugins
- Normal Post Request with Redirection
- Ajax Post Request without Reloading the Page
Normal Post Request with Redirection
jQuery(document).ready(function($) {
// Custom From submistion
jQuery(function($) {
$('#cst-jsbtn').click(function() {
var name2 = document.forms["cst-jsform"]["name"].value;
var name = document.getElementById("name").value;
if (name == "" || name == null) {
alert("Name field must be filled!");
return false;
}
var email = document.forms["cst-jsform"]["email"].value;
var at_position = email.indexOf("@");
var dot_position = email.lastIndexOf(".");
if (at_position < 1 || dot_position < at_position + 2 || dot_position + 2 >= email.length) {
alert("Given email address is not valid.");
return false;
}
var phone = document.forms["cst-jsform"]["phone"].value;
if (phone == "" || phone == null) {
if (!isNaN(phone)) {
alert("Please enter correct phone number.");
}
return false;
}
var state = document.forms["cst-jsform"]["state"].value;
if (state == "0" || state == null) {
alert("Please select a state.");
return false;
}
document.forms["cst-jsform"].submit();
});
//end cstform
});
// end Custom From submistion
});
// Ajax Post Request without Reloading the Page
jQuery(document).ready(function($) {
// Custom From submistion
jQuery(function($) {
$('#cst-jsbtn').click(function() {
var name2 = document.forms["cst-jsform"]["name"].value;
var name = document.getElementById("name").value;
if (name == "" || name == null) {
alert("Name field must be filled!");
return false;
}
var email = document.forms["cst-jsform"]["email"].value;
var at_position = email.indexOf("@");
var dot_position = email.lastIndexOf(".");
if (at_position < 1 || dot_position < at_position + 2 || dot_position + 2 >= email.length) {
alert("Given email address is not valid.");
return false;
}
var phone = document.forms["cst-jsform"]["phone"].value;
if (phone == "" || phone == null) {
if (!isNaN(phone)) {
alert("Please enter correct phone number.");
}
return false;
}
var state = document.forms["cst-jsform"]["state"].value;
if (state == "0" || state == null) {
alert("Please select a state.");
return false;
}
var data = $('#cst-jsform').serialize();
var url = window.location.href;
data += "&url=" + url;
$.ajax({
url: "https://www.example.com/customer-information.php",
type: "POST",
data: data,
complete: function(json) {
var jsonData = $.parseJSON(json.responseText);
if (jsonData['msg'] == 'success') {
$('#jsform-success').show();
$('#jsform-success').text('Thank you message!');
$("#cst-jsform").hide();
} else {
alert("Sorry! Something went wrong. Please try again.");
}
}
});
});
//end cstform
$('#cstpop-jsbtn').click(function() {
var name = document.forms["cstpop-jsform"]["name"].value;
if (name == "" || name == null) {
alert("Name field must be filled!");
return false;
}
var email = document.forms["cstpop-jsform"]["email"].value;
var at_position = email.indexOf("@");
var dot_position = email.lastIndexOf(".");
if (at_position < 1 || dot_position < at_position + 2 || dot_position + 2 >= email.length) {
alert("Given email address is not valid.");
return false;
}
var phone = document.forms["cstpop-jsform"]["phone"].value;
if (phone == "" || phone == null) {
if (!isNaN(phone)) {
alert("Please enter correct phone number.");
}
return false;
}
var state = document.forms["cstpop-jsform"]["state"].value;
if (state == "0" || state == null) {
alert("Please select a state.");
return false;
}
var data = $('#cstpop-jsform').serialize();
var url = window.location.href;
data += "&url=" + url;
$.ajax({
url: "https://www.exmaple.com/customer-information.php",
type: "POST",
data: data,
complete: function(json) {
var jsonData = $.parseJSON(json.responseText);
if (jsonData['msg'] == 'success') {
$('#pop-success').text('Succes Message!');
setTimeout(function() {
PUM.close(31851);
}, 2000);
} else {
alert("Sorry! Something went wrong. Please try again.");
}
}
});
});
//end cstpop
});
// end Custom From submistion
});
Create PHP function to submit form fields into Database Table.
So for the final part we will create a Database table and create a php function to proccess to Contact Form for WordPress.
Database Table
CREATE TABLE `wp_customer_information` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(45) COLLATE utf8mb4_unicode_520_ci NOT NULL,
`email` varchar(45) COLLATE utf8mb4_unicode_520_ci NOT NULL,
`phone` varchar(45) COLLATE utf8mb4_unicode_520_ci NOT NULL,
`state` varchar(45) COLLATE utf8mb4_unicode_520_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_520_ci;
PHP Function
Create a php file and add a function to insert contact form input into database table. You can save the file in the project directory or where ever you are able to access using the url.
<?php
// Get data
$name = $_POST["name"];
$email = $_POST["email"];
$phone = $_POST["phone"];
$state = $_POST["state"];
require_once ('wp-config.php');
if ('POST' != $_SERVER['REQUEST_METHOD'])
{
$protocol = $_SERVER['SERVER_PROTOCOL'];
if (!in_array($protocol, array(
'HTTP/1.1',
'HTTP/2',
'HTTP/2.0'
)))
{
$protocol = 'HTTP/1.0';
}
header('Allow: POST');
header("$protocol 405 Method Not Allowed");
header('Content-Type: text/plain');
exit;
}
else
{
if ($name != NULL && $email != NULL && $phone != NULL && $state != NULL)
{
// Database connection
$conn = mysqli_connect("localhost", "database_user", "database_password", "database");
if (!$conn)
{
die('Problem in database connection: ' . mysql_error());
}
// Data insertion into database
$query = "INSERT INTO `database`.`table_name` (name,email,phone,state) VALUES ('$name','$email','$phone','$state')";
mysqli_query($conn, $query);
$output = array(
"msg" => "success",
);
}
else
{
$output = array(
"msg" => "error",
);
}
echo json_encode($output);
}
// var_dump($query);
// exit();
// Redirection to the success page
// header("Location: https://www.vannstudios.com/");
?>
If you want to redirect to a custom page uncomment the redirection part and comment echo. So that you can show a customised success page at the end.
Top WordPress Plugins for Contact Form Builders
1. Contact Form Builder - By wpdevart
Active installations: 9,000+
2. Contact Form 7 - By Takayuki Miyoshi
Active installations: 5+ million
3. Contact Form by WPForms
Active installations: 1+ million